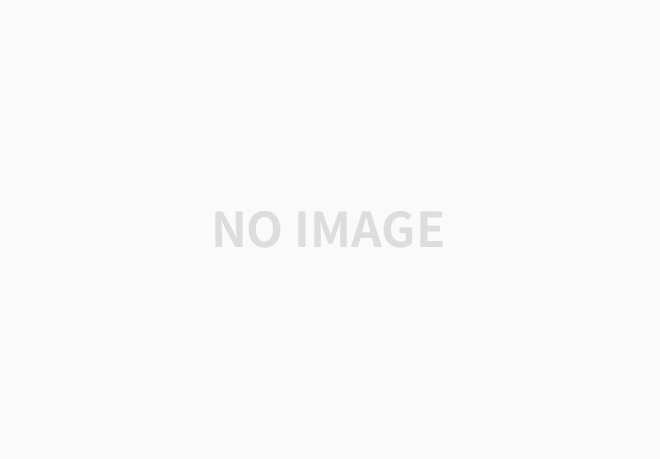
매우 쉬운 문제이다!
수는 중복되지 않는다는 점에서 HashSet을 안쓰고 ArrayList or 배열로 풀 수 있다고 판단했다!
로직은 쉽기 때문에 넘어가겠다! 이번에는 정렬에 기본을 쌓기 위해 3가지 문제를 풀었다!
1. ArrayList
2. 배열
3. Hash Set
[최종코드 - ArrayList]
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(br.readLine());
ArrayList<Integer> arr = new ArrayList<>();
for (int i = 0; i < n; i++)
arr.add(Integer.parseInt(br.readLine()));
Collections.sort(arr);
for (int num : arr)
System.out.println(num);
}
}
[최종코드 - 배열]
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(br.readLine());
int[] arr = new int[];
for (int i = 0; i < n; i++)
arr[i] = Integer.parseInt(br.readLine());
Arrays.sort(arr);
for (int num : arr)
System.out.println(num);
}
}
[최종코드 -Hash Set]
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(br.readLine());
HashSet<Integer> set = new HashSet<>();
for (int i = 0; i < n; i++)
set.add(Integer.parseInt(br.readLine()));
int[] arr = set.stream()
.sorted()
.mapToInt(Integer::intValue)
.toArray();
for (int num : arr)
System.out.println(num);
}
}
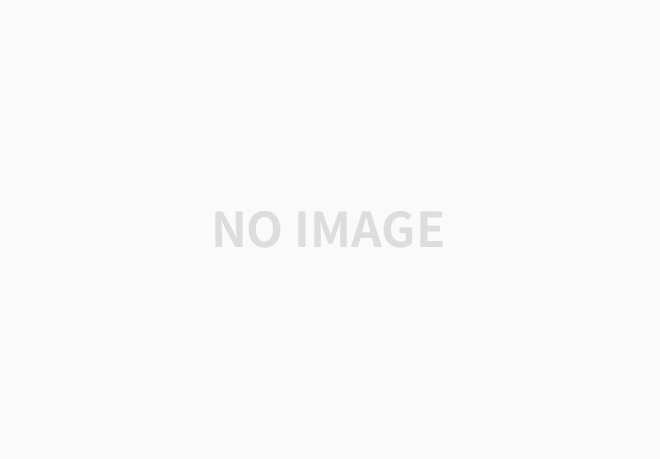
배운점
ArrayList와 배열의 Sort 방법이 좀 달라서 서치를 좀 했다. 좋은 공부가 되었다!
'코딩테스트 > 백준' 카테고리의 다른 글
[백준 JAVA] 25305 - 커트라인 (0) | 2024.12.08 |
---|---|
[백준 JAVA] 2587 - 대표값2 (0) | 2024.12.08 |
[백준 JAVA] 2839 - 설탕 배달 (1) | 2024.12.06 |
[백준 JAVA] 2798 - 블랙잭 (2) | 2024.12.05 |
[백준 JAVA] 1929 - 소수 구하기 (0) | 2024.12.04 |